Показ последних сообщений форума
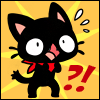
alexander.egorov
Модератор
12 апреля 2013 г.
1. Размещаем код в коде ТДС форума или создаем файл \modules\forum\last\posts\show.php
2. Вызов в макете:
<?php
if (Core::moduleIsActive('forum'))
{
$oForum = Core_Entity::factory('Forum', 1);
$Forum_Last_Posts_Show = new Forum_Last_Posts_Show(
$oForum
);
$Forum_Last_Posts_Show
->xsl(
Core_Entity::factory('Xsl')->getByName('ПоследниеСообщенияФорума')
)
->show();
}
?>
3. Код XSL-шаблона "ПоследниеСообщенияФорума"
<?php
/**
* Показ последних сообщений форума.
*
* <code>
* $oForum = Core_Entity::factory('Forum', 1);
*
* $Forum_Last_Posts_Show = new Forum_Last_Posts_Show(
* $oForum
* );
*
* $Forum_Last_Posts_Show
* ->xsl(
* Core_Entity::factory('Xsl')->getByName('ПоследниеСообщенияФорума')
* )
* ->show();
* </code>
*
* @package HostCMS 6\Forum
* @version 6.x
* @author Hostmake LLC
* @copyright © 2005-2013 ООО "Хостмэйк" (Hostmake LLC),http://www.hostcms.ru
*/
class Forum_Last_Posts_Show extends Core_Controller
{
/**
* Allowed object properties
* @var array
*/
protected $_allowedProperties = array(
'limit',
);
protected $_forumTopicPosts = NULL;
/**
* Constructor.
* @param Forum_Model $oSiteuser user
*/
public function __construct(Forum_Model $oForum)
{
parent::__construct($oForum->clearEntities());
$this->limit = 3;
$this->_forumTopicPosts = Core_Entity::factory('Forum_Topic_Post');
$this->_forumTopicPosts
->queryBuilder()
->select('forum_topic_posts.*')
->join('forum_topics', 'forum_topics.id', '=', 'forum_topic_posts.forum_topic_id')
->join('forum_categories', 'forum_categories.id', '=', 'forum_topics.forum_category_id')
->join('forum_groups', 'forum_groups.id', '=', 'forum_categories.forum_group_id')
->where('forum_groups.forum_id', '=', $oForum->id)
->where('forum_groups.deleted', '=', 0)
->where('forum_categories.deleted', '=', 0)
->where('forum_topics.deleted', '=', 0)
->clearOrderBy()
->orderBy('forum_topic_posts.datetime', 'DESC')
->limit($this->limit);
}
public function forumTopicPosts()
{
return $this->_forumTopicPosts;
}
/**
* Show built data
* @return self
*/
public function show()
{
$oForum = $this->getEntity();
$aForum_Topic_Posts = $this->_forumTopicPosts->findAll(FALSE);
foreach ($aForum_Topic_Posts as $oForum_Topic_Posts)
{
$this->addEntity(
$oForum_Topic_Posts->clearEntities()
->showXmlSiteuser(TRUE)
);
}
return parent::show();
}
}
?>
/**
* Показ последних сообщений форума.
*
* <code>
* $oForum = Core_Entity::factory('Forum', 1);
*
* $Forum_Last_Posts_Show = new Forum_Last_Posts_Show(
* $oForum
* );
*
* $Forum_Last_Posts_Show
* ->xsl(
* Core_Entity::factory('Xsl')->getByName('ПоследниеСообщенияФорума')
* )
* ->show();
* </code>
*
* @package HostCMS 6\Forum
* @version 6.x
* @author Hostmake LLC
* @copyright © 2005-2013 ООО "Хостмэйк" (Hostmake LLC),
*/
class Forum_Last_Posts_Show extends Core_Controller
{
/**
* Allowed object properties
* @var array
*/
protected $_allowedProperties = array(
'limit',
);
protected $_forumTopicPosts = NULL;
/**
* Constructor.
* @param Forum_Model $oSiteuser user
*/
public function __construct(Forum_Model $oForum)
{
parent::__construct($oForum->clearEntities());
$this->limit = 3;
$this->_forumTopicPosts = Core_Entity::factory('Forum_Topic_Post');
$this->_forumTopicPosts
->queryBuilder()
->select('forum_topic_posts.*')
->join('forum_topics', 'forum_topics.id', '=', 'forum_topic_posts.forum_topic_id')
->join('forum_categories', 'forum_categories.id', '=', 'forum_topics.forum_category_id')
->join('forum_groups', 'forum_groups.id', '=', 'forum_categories.forum_group_id')
->where('forum_groups.forum_id', '=', $oForum->id)
->where('forum_groups.deleted', '=', 0)
->where('forum_categories.deleted', '=', 0)
->where('forum_topics.deleted', '=', 0)
->clearOrderBy()
->orderBy('forum_topic_posts.datetime', 'DESC')
->limit($this->limit);
}
public function forumTopicPosts()
{
return $this->_forumTopicPosts;
}
/**
* Show built data
* @return self
*/
public function show()
{
$oForum = $this->getEntity();
$aForum_Topic_Posts = $this->_forumTopicPosts->findAll(FALSE);
foreach ($aForum_Topic_Posts as $oForum_Topic_Posts)
{
$this->addEntity(
$oForum_Topic_Posts->clearEntities()
->showXmlSiteuser(TRUE)
);
}
return parent::show();
}
}
?>
2. Вызов в макете:
<?php
if (Core::moduleIsActive('forum'))
{
$oForum = Core_Entity::factory('Forum', 1);
$Forum_Last_Posts_Show = new Forum_Last_Posts_Show(
$oForum
);
$Forum_Last_Posts_Show
->xsl(
Core_Entity::factory('Xsl')->getByName('ПоследниеСообщенияФорума')
)
->show();
}
?>
3. Код XSL-шаблона "ПоследниеСообщенияФорума"
<?xml version="1.0" encoding="utf-8"?>
<!DOCTYPE xsl:stylesheet>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:hostcms="http://www.hostcms.ru/"
exclude-result-prefixes="hostcms">
<xsl:output xmlns="http://www.w3.org/TR/xhtml1/strict" doctype-public="-//W3C//DTD XHTML 1.0 Strict//EN" encoding="utf-8" indent="yes" method="html" omit-xml-declaration="no" version="1.0" media-type="text/xml"/>
<xsl:template match="/forum">
<style>
.posts_list dt
{
background: url("/images/li.gif") no-repeat scroll 0 8px transparent;
color: #606060;
font-weight: bold;
margin: 0 0 7px;
padding-left: 15px;
}
.posts_list dd
{
margin: 0;
}
.user {
color: #AAAAAA;
font-size: 9pt;
margin: 5px 0 0;
}
.user span
{
padding-right: 15px;
}
</style>
<p class="h1">Последние сообщения</p>
<dl class="posts_list">
<!-- Выбираем посты -->
<xsl:apply-templates select="forum_topic_post" />
</dl>
</xsl:template>
<xsl:template match="forum_topic_post">
<dt>
<!-- Дата и время -->
<xsl:value-of disable-output-escaping="yes" select="datetime"/>
</dt>
<dd>
<!-- Текст -->
<xsl:value-of disable-output-escaping="yes" select="text"/>
</dd>
<p class="user">
<!-- Логин -->
<img src="/images/user.png" />
<span><xsl:value-of disable-output-escaping="yes" select="siteuser/login"/></span>
</p>
</xsl:template>
</xsl:stylesheet>
<!DOCTYPE xsl:stylesheet>
<xsl:stylesheet version="1.0"
xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:hostcms="http://www.hostcms.ru/"
exclude-result-prefixes="hostcms">
<xsl:output xmlns="http://www.w3.org/TR/xhtml1/strict" doctype-public="-//W3C//DTD XHTML 1.0 Strict//EN" encoding="utf-8" indent="yes" method="html" omit-xml-declaration="no" version="1.0" media-type="text/xml"/>
<xsl:template match="/forum">
<style>
.posts_list dt
{
background: url("/images/li.gif") no-repeat scroll 0 8px transparent;
color: #606060;
font-weight: bold;
margin: 0 0 7px;
padding-left: 15px;
}
.posts_list dd
{
margin: 0;
}
.user {
color: #AAAAAA;
font-size: 9pt;
margin: 5px 0 0;
}
.user span
{
padding-right: 15px;
}
</style>
<p class="h1">Последние сообщения</p>
<dl class="posts_list">
<!-- Выбираем посты -->
<xsl:apply-templates select="forum_topic_post" />
</dl>
</xsl:template>
<xsl:template match="forum_topic_post">
<dt>
<!-- Дата и время -->
<xsl:value-of disable-output-escaping="yes" select="datetime"/>
</dt>
<dd>
<!-- Текст -->
<xsl:value-of disable-output-escaping="yes" select="text"/>
</dd>
<p class="user">
<!-- Логин -->
<img src="/images/user.png" />
<span><xsl:value-of disable-output-escaping="yes" select="siteuser/login"/></span>
</p>
</xsl:template>
</xsl:stylesheet>
Вы только что начали читать предложение, чтение которого вы уже заканчиваете.

mjoey
25 апреля 2013 г.
А как вывести три последних темы форума?
Авторизация